Variables are normally declared with a $
token at the start of them,
as with PHP, Perl, Bash and many other scripting languages, or as of ZPE 1.9.3/YASS 21
declared using the let
keyword in which case they can
be unbound from the $
token.
Variables should not be keywords, e.g.
$is
or $list
are
not valid names for variables.
$a = 43 $b = true $c = false $d = "Hello" $e = $a || $b $f = $c
Version 1.5.3 introduced the variable subscript syntax but it should be avoided before version 1.6.3 as it was buggy and didn't always produce expected results.
$a = [43, 44, 45] print($a[1])
Unbound assignment and variables
Unbound assignment is a form of assignment where variables are defined and assigned without any
declaration. In ZPE/YASS prior to version 1.9.3 and PHP for example, a variable was
bound to a $
.
Following the update made in ZPE 1.9.3, variables could be unbound from the $
symbol and defined freely (see Using the let keyword in ZPE) using
the let
- or var
keywords.
Following a small update, version 1.11.8 was the first version to allow variable declaration without
the use of the $
symbol, the let
keyword or the var
keyword prior to it:
$x = 10 print($x)
This is done with compiler assumption and it's part of the complex processes the compiler carries out.
Using the let keyword in ZPE
Using the let
keyword in ZPE 1.9.3+/YASS 21+ you can easily assign variables:
let $x = 10 print($x)
ZPE 1.9.3 (Hawick) brought one of the most desired features to ZPE/YASS - variable
names that do not need preceeded by a $
sign.
It does this without using any late inference to determine variables and does it during
compile time. It's important to note that the
create_variable
function creates variables that have the $
sign
at the start of them since those variables cannot effectively be checked at compile time.
let x = 10 print(x)
This also works where variables can be assigned, e.g. in a for loop:
for (let x = 0 to 10) print(x) end for
let
keyword can also be substituted with the var
keyword,
making the syntax more like ES6 and JavaScript.
Using the be keyword
As of version 1.9.6 (St Boswells), it is now possible to declare a variable
using the be
keyword. This is a user friendly alternative
way of saying set the value to.
let x be 10
Assignment by reference
ZPE 1.10.4 (Exceptional Elephant) brought full by reference to ZPE. This is a memory efficient method of using variables compared with the alternative.
By reference works on ZPETypes, which includes strings, objects, lists, maps and
functions. By reference is signified by the &$
symbol,
e.g. &$x
.
$x = "Hello world" $y = &$x $z = $x $x[3] = "p" //Will print Helpo world print($x) //Will print Helpo world print($y) //Will print Hello world print($z)
Previous versions of ZPE always used by reference, since ZPETypes were introduced.
So for example $x = $y
would make $x
reference $y
. Also, the reference
only happens in the assignment as of version 1.10.4, but will come to parameter
passing soon.
Non-standard values
Version 1.5.0 added support for hexadecimal and octal values straight from the compiler. The compiler works these out and transforms them to their integer equivalents during compile time.
The following sample shows this:
$hex = 0x43 $octal = 0o33
Hexadecimal numbers start with a 0 (zero) followed by an x followed by an hexadecimal values.
Octal numbers start with a 0 (zero) followed by an o and then octal numbers.
These styles were borrowed from C.
Data types and TYPO
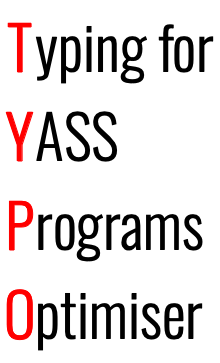
ZPE 1.6.7 adds support for typing through the TYPO (Typing for YASS Programs Optimiser) typing system. TYPO is a runtime-based typing system designed for educational and learning use but also to add stricter programming practices to ZPE. As of early 2022, the standard library is now entirely written using strong typing and requires TYPO to function.
ZPE has 8 primitive types as of version 1.6.7 (prior to that it had several others, but most have since been combined into the object syntax such as the image type). Although there are 8 underlying primitive types there are only 6 different ways to declare them. The types and their declarative keywords are shown below:
The types are as follows:
Data type | Keyword | Example | Purpose |
---|---|---|---|
Mixed | - |
$x = "Hello"
$x = 10
|
To hold a value of any data type and be dynamic in it's type. |
String |
string
|
string x = "Hello"
|
To hold text or any character from the keboard. |
Integer |
number
|
number x = 10
|
To hold a numerical value |
Real |
number x = 10.3
|
||
Boolean |
boolean
|
boolean x = true
|
To hold a true or false value |
List |
list
|
list x = [1, 2, 3, 4]
|
To hold a list, array, map or ordered map |
Map |
list x = [1 => 99, 2 => 98, 3 => 97]
|
||
Function |
function
|
function x = function () print ("Hello") end function
|
To hold a reference to a function or an actual anonymous function |
Object |
object
|
object x = {name : "Pete"}
|
To hold an object value, which itself can contain other properties. |
ZPE does not distinguish the difference between an integer value and a double value until assignment has taken place. The same goes for lists and maps, which both come under the List type but are represented differently in memory.
When setting the data type of a variable as of version 1.9.7 (Galashiels, July 2021),
the declare
keyword is used:
declare $a as string = "Hello" declare $b as number = 10 declare $c as number = 10.5 declare $d as boolean = true declare $e as list = ["Hello", "World"] declare $f as list = ["Hello" => "World"] declare $g as function = function() { print("Hello world") } declare $h as object = {name : "Jamie"}
Further to this, ZPE 1.9.7 (Galashiels, July 2021) adds support for C-style type declarations:
string $a = "Hello" number $b = 10 number $c = 10.5 boolean $d = true list $e = ["Hello", "World"] list $f = ["Hello" => "World"] function $g = function() { print("Hello world") } object $h = {name : "Jamie"}
ZPE 1.9.7 (Galashiels, July 2021) also permits the definition of types within a structure/class:
structure testerprivate number val1 = 10private declare val2 as number = 10public function setter($v) this->val1 = $v end function public function getter() return this->val1 end function end structure function main() $x = new tester() print($x->getter()) $x->setter(15) print($x->getter()) end function
As with all built-in ZPE keywords, types and the declare
and as
keywords are all lowercase when using the case sensitive compiler.
Using the declare
keyword, variables can also be unbound from
the $
token but they must be specified with a data type
whenever the declare
keyword is used.
declare a as string = "Hello" declare b as number = 10 declare c as number = 10.5 declare d as boolean = true declare e as list = ["Hello", "World"] declare f as list = ["Hello" => "World"] declare g as function = function() { print("Hello world") } declare h as object = {name : "Jamie"}
Inline assignment
Version 1.6.7 also added inline assignment which was originally thought to be a complicated procedure but ended up being relatively simple. This syntax allows declarations to take place within other expressions, e.g. an if statement:
$f = new SequentialFile() $f->open_file("list.txt") while (($check = $f->has_next()) == true) print($f->read_line()) end while
Notice that the assignment is bracketed! This is so important to prevent the compiler parsing it as a boolean assignment.
$x = get_input("Insert a value.") if (($v = $x + 3) == 10) print($v) end if
$l = [11, 22, 33, 44, 55, 66, 77] $i = 0 $search = 33 //Find 33 in the list while (($current = $l[$i]) != $search) $i++ end while print($i)
This type of assignment was added in ZPE 1.6.7 and improved over time.
Destructuring assignment
ZPE 1.12.6 (Kilted Kelpie, June 2024) brought in destructured assignment, in other words variables can be assigned individually to parts or elements of a set, tuple, list, record or object.
($x, $y, $z) = [1, 2, 3]
This will result in three variables being created ($x
,
$y
, $z
) with a value from each position in
the list.
Multiple assignments
Actually seen as an odd side effect of the compiler (and I'm not entirely sure which version added support for it as of yet), multiple assignment in ZPE/YASS is very easy:
$w = $x = $y = $z = 10 print($w) print($x) print($y) print($z)
As mentioned above, I am not sure which version this was added in since it is a side-effect of some other compiler feature and was not intentional.
Variable name tester
Use this form to test a variable name.