What is an array?
Put simply, an array is a collection of other items. In programming, an array can be seen to similar to the way that variables are stored in memory. In this sense, there is a direct mapping between the variable name or index and the value.
Arrays are incredibly useful ways of storing lots of information in one easy to access place. Not only this, unlike 1-dimension variables (all variables used in this tutorial), array values can be defined at runtime.
As mentioned, arrays can be seen as maps:
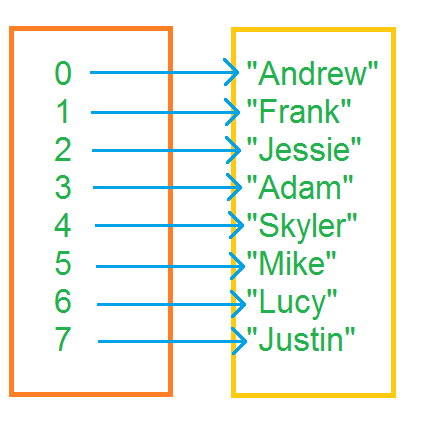
An array provides a map from the left (index or key) to something on the right (value)
Arrays in JavaScript
Declaring arrays
In JavaScript, arrays are dynamically sized, meaning that the size of the array can change size at any point of time.
An array is declared as follows:
var array = [];
This defines an empty array. An array does not need to be empty to start with, as shown below an array can contain elements upon instantiation:
var names = ['Jamie', 'Balfour'];
Adding elements to an array
Adding elements to the array can be done in two ways. The first of those is to simply specify an index for the element and assign a value to it:
var names = ['Jamie', 'Michael']; names[2] = 'David'; alert(names);
It is important to note that the first element of an array is at index 0 in JavaScript.
The alternative to adding an element this way is to consider the array as a stack structure, whereby everything follows a first in first out system:
var names = ['Jamie', 'Michael']; names.push('David'); alert(names);
Retrieving elements from an array
JavaScript defines a simple way of getting a value from an array. JavaScript like many languages uses the index (a numeric location in the array) to retrieve a value from an array:
var names = ['Jamie', 'Michael']; alert(names[1]);
Getting the size of an array
In JavaScript an array is an object type and has properties and methods. One such property is the size or
length
of the array. This allows you to check how many elements there are in an array:
var names = ['Jamie', 'Michael', 'David']; alert(names.length);