Borders
Borders are a good way of separating individual elements. They are useful in visual design.
Borders work much like margins and padding on an element in that they are given to each individual side of an element.
.borders{ border-top-width: 2px; border-top-color: #f60; border-top-style: solid; }
The first thing to note is that this affects only the top border as demonstrated below:
However, this method is slow and tedious because it takes a total of three properties to modify the top border. This can again be shorthanded to:
.borders{
border-top: 10px #ddd solid;
}
This along with the first example can be applied to each of the four sides of an element as shown below:
.borders{ border-top: 5px #f60 solid; border-bottom: 5px #f60 solid; border-left: 5px #f60 solid; border-right: 5px #f60 solid; }
This gives all four sides the same border, or it can be customised by changing each property individually.
However, this is still a big range of properties, particularly if all four sides are intended to have the same border. CSS has another shorthand that abstracts over this even further:
.borders{
border: 5px #f60 solid;
}
Also, using border
property and then using border-bottom
after it will overwrite the border
on the bottom of
the element.
Just as with padding and margins, adding a border to an element adds to the size. If an element has a width set to 100% and a border of 5px then it's width is calculated as 100% + 5px. Again specifying no width on a block element and adding a border gives it an automatic width of 100% - 5px when a border of 5px is applied to the element.
The border
property can also be given styles. In the previous examples, only the solid
style
has been given. This table is a concise list of all the attributes that can be used instead of solid
as the border-style
:
Value | Example |
---|---|
solid |
Solid
|
dashed |
Dashed
|
dotted |
Dotted
|
double |
Double
|
groove |
Groove
|
ridge |
Ridge
|
inset |
Inset
|
outset |
Outset
|
Box shadows
As of CSS3, elements can be given a shadow around them. Because CSS3 is was still in Draft status when Internet Explorer 8 was released, support for it in browsers like Internet Explorer 8 and early versions of Chrome, Firefox, Opera and Safari had no support or limited support. There is no way of adding this to browsers with no support but for those with limited support, there does exist prefixed supportPrefixed support Prefixed support refers to putting the vendor prefix at the front of the property. This is done because the way the vendor has implemented some new property is not following the requirements of the specification and so is implemented as the vendor wishes.. There is more in the article in this tutorial on Cross platform and browser compatibility.
Box shadows can be achieved with the box-shadow
property.
As with most CSS properties, the box-shadow
property can be given the values initial
, inherit
, none
.
As well as these three values, it can of course be given a value with meaning. They are described as:
.shadowed{
box-shadow:horizontal_position vertical_position [blur_distance size_of_shadow shadow_colour inset]
}
The horizontal_position sub-value is an size (such as pixels or percentage) that describes the shadow location from the left of the element it is applied to. The vertical_position sub-value is a size that describes the shadow location from the top of the element it is applied to. Both of these sub-values are essential in this value.
The blur_distance sub-value is also a size, but this describes the distance as to which the blur should stretch from the edges, for instance, if given 15px it would stretch further than (the default) 5px.
The size_of_shadow sub-value again is also an size and describes the size of the actual shadow. This will look like a solid block if the blur_distance is very small.
The shadow_colour sub-value represents the colour of the shadow. By default is black or #000.
The inset value states whether the shadow should go inside the element, so instead of acting like a shadow, it acts like it's inside the element (as if the element had high walls, and they shadowed into it).
The following is an example of box shadowing:
.shadowed{
box-shadow:2px 2px 5px 4px #f60;
}
The following is a what this sample would look like:
The following sample demonstrates shadowing going inside:
.shadowed{
box-shadow:0px 0px 2px 1px #aaa inset;
}
Again, this sample shows exactly what the above the sample would like:
Internal box shadows are useful design features and are found everywhere.
Border radius
Border radii are used to define the curve of the borders on an element. Such examples of curved elements on this website include the search box and the main logo on the menu.
The border-radius
property can be used in a few different ways. The first is the long way, that applies the border-radius
to each side:
.border-radius{ border-top-left-radius: 10px; border-top-right-radius: 35px; border-bottom-left-radius: 35px; border-bottom-right-radius: 10px; }
This gives the following results:
Of course doing it this way is slow and takes a long time (it is precise, and easier to remember than the next example), so a CSS shorthand was developed:
.border-radius{
border-radius:10px 35px;
}
This gives the exact same results:
The problem is that because this form is shorter, it is less precise. For instance:
.border-radius{ border-top-left-radius: 10px; border-top-right-radius: 35px; border-bottom-left-radius: 10px; border-bottom-right-radius: 35px; }
The example would look like this:
This cannot be represented in two values, so instead the full border-radius
shorthand property is expanded as shown:
.border-radius{
border-radius:top-left-radius top-right-radius bottom-left-radius bottom-right-radius;
}
This provides a shorthand solution for a larger problem. The final shorthand CSS for border-radius
is shown below:
.border-radius{
border-radius:all-border-radius;
}
This applies the curved edge to all four sides of the element.
Circlular elements
Most elements have a very basic square design, but it is possible to make an element circular, to the extent that it is a true circle (not even an oval). To achieve this, the element needs to have its height and width equal.
The border-radius
property can then be applied with a non-fixed size. It utilises the last shorthand shown.
The element itself will still be rendered as a square, but it will look like a perfect circle to a user.
.circular-element{ width:150px; height:150px; border-radius:50%; }
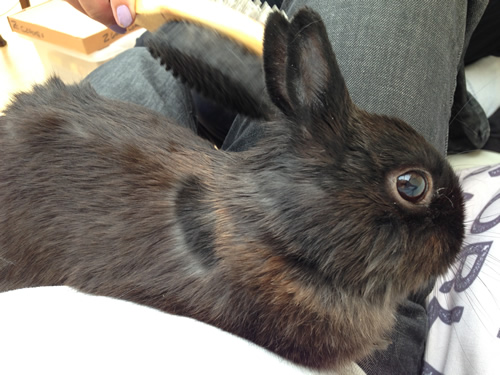
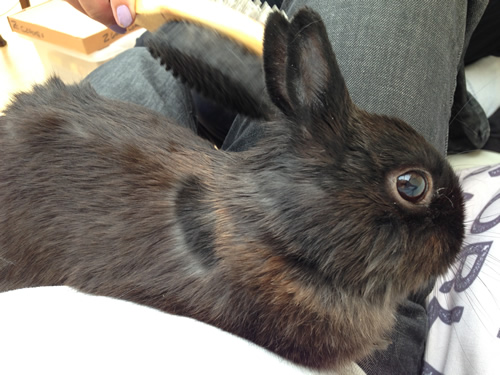
Box-sizing
Box sizing is used to inform the browser how to interpret size properties. It can be used to fix a lot of problems in browsers. For instance:
The code for this sample is shown below:
<div style="width:300px; margin:auto;"> <textarea style="width:100%; padding:15px;"></textarea> </div>
This tutorial has already mentioned that an element if an element is given a width of 100% and given padding of 15px it will stretch some 15px out of it's parent. This is shown above in the above example.
The code for this sample is shown below:
<div style="width:300px; margin:auto;"> <textarea style="display:block;"></textarea> </div>
However, it was also mentioned that an element with display: block
will stretch
the width of it's entire parent. This however, is not the case with the form <textarea>
in the above
example.
Not only that, if it manages to leave it's parent, adding padding makes this situation worse.
The fix is the box-sizing
property. By giving an element such as a <textarea>
a box-sizing
of border-box the element considers it's width plus padding into the equation:
Now using the first example and the new box-sizing
property, the <textarea>
fits nicely:
<div style="width:300px; margin:auto;"> <textarea style="width:100%; padding:15px; box-sizing:border-box;"></textarea> </div>