An error can occur in any program. Most programs know how to deal with this so that the user's experience is not disrupted by an error message.
Try...Catch...End Try
The Try...Catch...End Try statement is often better known simple as a Try statement. This piece of code cleverly wraps other code and in the off chance that there is an error in the code, the Catch section of the Try statement is followed.
Try 'Code to try and run Catch ex As Exception 'Code for when an error occurs End Try
The theory of a Try statement is that if an error occurs due to a user input, the program can prevent further execution. It's purpose is not for
preventing program faults such as trying to start a program that does not exist.
The following sample would normally cause an error due to the arithmetic overflow of 2019443 * 2019443 *
8 being larger than 32 bits in binary (the size of an Integer
).
Dim i As Integer = 2019443 MsgBox(i * i * 8)
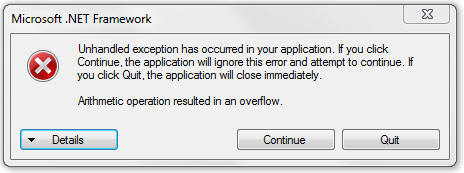
This is called a runtime time error and these can be incredibly annoying for end users of a program. The .NET collection of languages all feature an error catching tool which allows users to continue what they are doing so that they can save or whatever else they may need to do. None the less, it should be avoided. The following sample shows how to get the message with information about the error out.
Dim i As Integer = 2019443 Try MsgBox(i * i * 8) Catch ex As Exception MsgBox(ex.Message) End Try
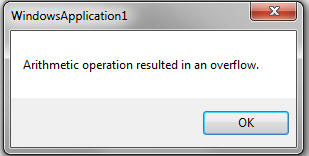
The information about the error is much easier to read here, so if the end user can understand some basic programming concepts this kind of dialog is much shorter and easier to understand.
Of course, the program does not need to output the error at all. It could ignore the error or it could follow a different path. The first of the following sample demonstrates a program following a different path when it encounters an error and the second sample demonstrates ignoring an error altogether.
Dim i As Integer = 2019443 Try MsgBox(i * i * 8) Catch ex As Exception MsgBox(i * 2) End Try
Dim i As Integer = 2019443 Try MsgBox(i * i * 8) Catch ex As Exception End Try MsgBox("Thank you for using the multiply program")