Subprograms
Python, like many scripting syntaxes, has just one subprogram declaration format.
Often called a procedure, a function, or a subroutine, a subprogram is a division of code that is separated from the rest. A subprogram is a way of organising code to perform one particular function. For instance, (in an abstract example), a subprogram could be used to turn on a light bulb or to turn off a plug socket. When it is needed, it is run (called). The part that gives the subprogram a name and parameters is called the signature. Below is a simple diagram that explains the subprogram structure:
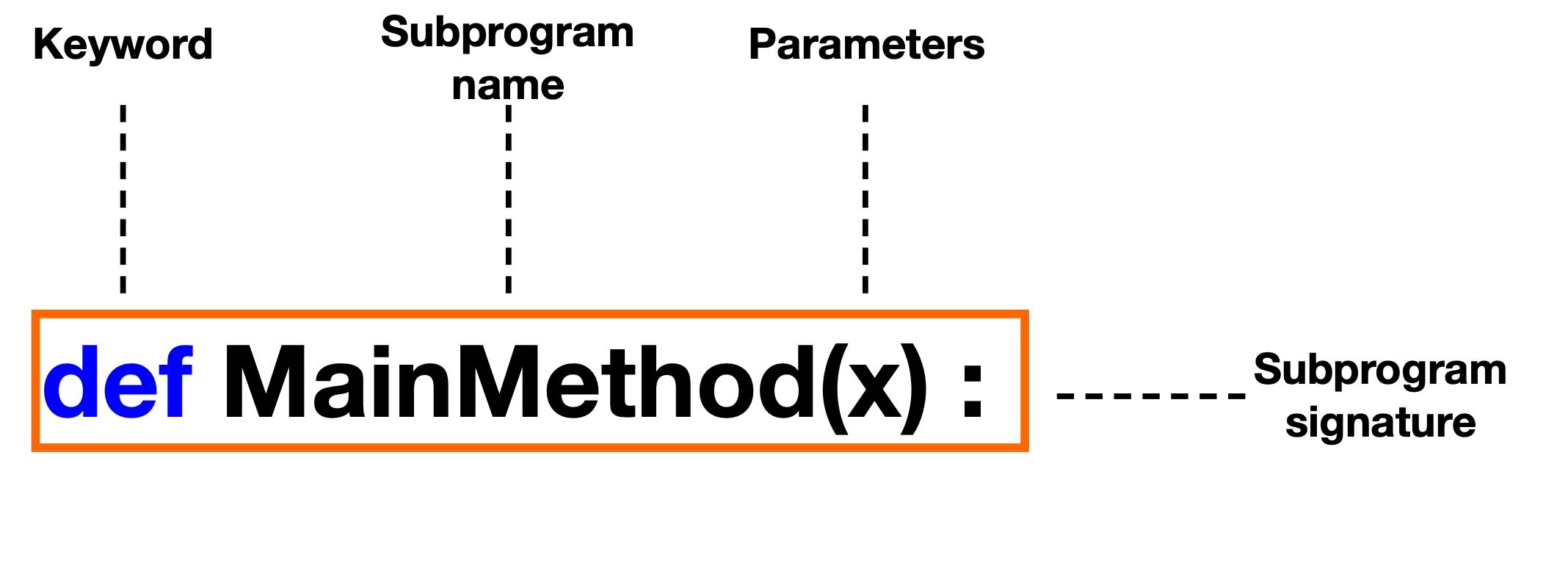
Defining a subprogram
Below is an example subprogram:
def main(): print ("Hello world")
Subprogram can however, return a value. If a subprogram returns a value, the value that it gives back can be used elsewhere.
Returning a value
A subprogram that returns, or gives back, a value is known as a function, since it's actions mimic that of a mathematical function:
A ⇒ B
In this example, A is an input the arrow is the process and B is the output. For every input there is exactly one output.
A function returning a value looks like the following sample:
def main(): return "Hello world"
With this, assigning the function call to a variable will give the variable the value
"Hello world"
.
Calling a subprogram
A subprogram can be called through a very simple syntax. Arguments are also given to subprogram calls within the brackets - these line up with parameters specified in the subprogram signature.
def main(): print ("Hello world") main()
The previous sample will call the subprogram named main. It will also provide no arguments to the call.
A subprogram with parameters can be called by inserting values in the positions of those parameters with arguments:
def add(n1, n2): print (n1 + n2) add(4, 7)
Recursion
Recursion is a big part of learning to program efficently and it is basically a subprogram calling itself over and over again until a termination condition (base case) is met. Recursion can be used to multiply two numbers by simply using addition.
def multiply(n1, n2, total):if n2 == 0:return n1 else: total = multiply(n1, n2 - 1, total) return total + n1 print(multiply(4, 3, 0))
Here is what happens in this program:
- multiply(4, 3, 0) calls multiply (4, 2, 0)
- multiply(4, 2, 0) calls multiply (4, 1, 0)
- multiply(4, 1, 0) calls multiply (4, 0, 0)
- multiply (4, 0, 0) then returns 0 + 4 (4) to multiply(4, 1, 0)
- multiply (4, 1, 0) then returns 4 + 4 (8) to multiply(4, 2, 0)
- multiply (4, 2, 0) then returns 8 + 4 (12) to multiply(4, 3, 0)
Recursion is something that to understand, one must first understand recursion. For more information on recursion, look at recursion.