Building a sidebar on a website can be challenging, especially if the sidebar is designed to stretch the whole container height.
There are many ways to construct a sidebar, and this article aims to cover just a few that work well.
A look at the issue
Currently, a lot of sidebars use the CSS float
property. This works well based on two things. The first is that the sidebar does not need to sit alongside another block element. If it does, the situation is complicated when the sidebar has more content than the main content.
Below is a sample of a floated sidebar:
The above sample has the following code:
<div style="background: green; padding: 5px 0"> <div style="float:left; background: #ddd; width:33%;"> <div style="padding:15px;"> This is the sidebar </div> </div> <div style="min-width:67%; background:#f0f0f0"> <div style="padding:15px;"> This is the main content </div> </div> </div>
If the same style of sidebar has a sidebar with more content than the main content, then there is a huge issue with the content of the sidebar not increasing the size of the owner container.
This is another line of text inside the sidebar.
The above sample has the following code:
<div style="background:green; padding: 5px 0"> <div style="float:left; background: #ddd; width:33%;"> <div style="padding:15px;"> This is the sidebar<br>This is another line of text inside the sidebar. </div> </div> <div style="min-width:67%; background:#f0f0f0"> <div style="padding:15px;"> This is the main content </div> </div> </div>
The thing to note is that in the sample the sidebar flows out with the container. This is the main problem with floating a sidebar.
The other main one occurs if you want the sidebar to stretch the height of the main content.
You can see it is a few lines long.
This means that the sidebar looks like it's just meant for the top, and it is just a floating element.
<div style="background:green; padding: 5px 0"> <div style="float:left; background: #ddd; width:33%;"> <div style="padding:15px;"> This is the sidebar<br> </div> </div> <div style="min-width:67%; background:#f0f0f0"> <div style="padding:15px;"> This is the main content.<br>You can see it is a few lines long.<br>This means that the sidebar looks like it's just meant for the top and it is just a floating element. </div> </div> </div>
A couple of solutions
There are many ways to implement the sidebar effectively.
The following samples use the following HTML:
<div style="background:green; padding: 5px 0" id="container"> <div id="sidebar"> <div style="padding:15px;"> This is the sidebar<br> </div> </div> <div id="main_content"> <div style="padding:15px;"> This is the main content.<br>You can see it is a few lines long.<br>This means that the sidebar looks like it's just meant for the top and it is just a floating element. </div> </div> </div>
1: Using JavaScript
The simplest solution to a whole collection of problems is always to use JavaScript. JavaScript for rendering should be avoided where possible, but it's one such solution to this problem (in this case, I am using jQuery to save time):
var sH = jQuery("#sidebar").height(); var mH = jQuery("#main_content").height(); var fH = 0; if(sH >= mH){ fH = sH; } else{ fH = mH; } jQuery("#sidebar").height(fH); jQuery("#main_content").height(fH);
You can see it is a few lines long.
This means that the sidebar looks like it's just meant for the top, and it is just a floating element.
Resizing this element will not affect it in the same way as before, but it is far from perfect since it fails on resize.
2: Using absolute positioning
Absolute positioning often fixes a variety of issues on modern browsers but messes up older browsers such as Internet Explorer 8.
I say this because absolute positioning is one of the easiest ways to make an element the exact size that you want and in the exact place that you want. This is why this solution works well for this problem.
This solution works well also because it forces the sidebar to stretch the height of the page and works with fixed-width sidebars and scalable sidebars (I used this solution for a while since it worked well).
The first step is to make the container element relative:
#container {
position: relative;
}
The next step is to make the sidebar position absolute and stretch from top to bottom. This example shows a right-hand sidebar:
#sidebar { position: absolute; top: 0; right: 0; bottom: 0; width: 320px; }
The final step is the main content. Leaving the main content as a full-width division, setting the right-hand margin will force it to ignore the sidebar:
#main_content {
margin-right: 320px;
}
This leaves space on the right-hand side for the sidebar. The sample below shows how this looks
You can see it is a few lines long.
This means that the sidebar looks like it's just meant for the top, and it is just a floating element.
So, whilst this solution works reasonably well, its biggest problem is when the sidebar is taller than the main content division.
One such solution is to set a minimum height on the main content division.
3: Using tables
This is just a solution, but you could nest everything in a table. I do not think this counts as a real-life solution for obvious reasons.
4: Using the table layout option
This is my favourite since CSS can make elements behave in the same manner as a table. This is the way I currently use on my website for many reasons:
- Both the main content and sidebar will be the same height
- There are no set heights on either the sidebar or main content
- The width of either or both of these elements may be fixed
- It is easy to switch to a responsive mode
- It is supported on older browsers reasonably well
Numbers 1 and 2 are the most crucial points here, particularly with websites like my own, where the main content and sidebar vary massively across different pages.
Most important is to set the layout to table and make sure it is fixed:
#container { display: table; table-layout: fixed; }
The next step is to make sure that the sidebar and main content are table cells:
#main_content, #sidebar { display: table-cell; } #sidebar { width: 320px; }
Below shows this in action:
You can see it is a few lines long.
This means that the sidebar looks like it's just meant for the top and it is just a floating element.
The greatest thing is the fact that it is compatible with browsers as far back as Internet Explorer 6.
Conclusion
There are many other options for building a sidebar, but some, like using a flexible grid, are not widely supported.
I stick by the fourth solution given as the best option.
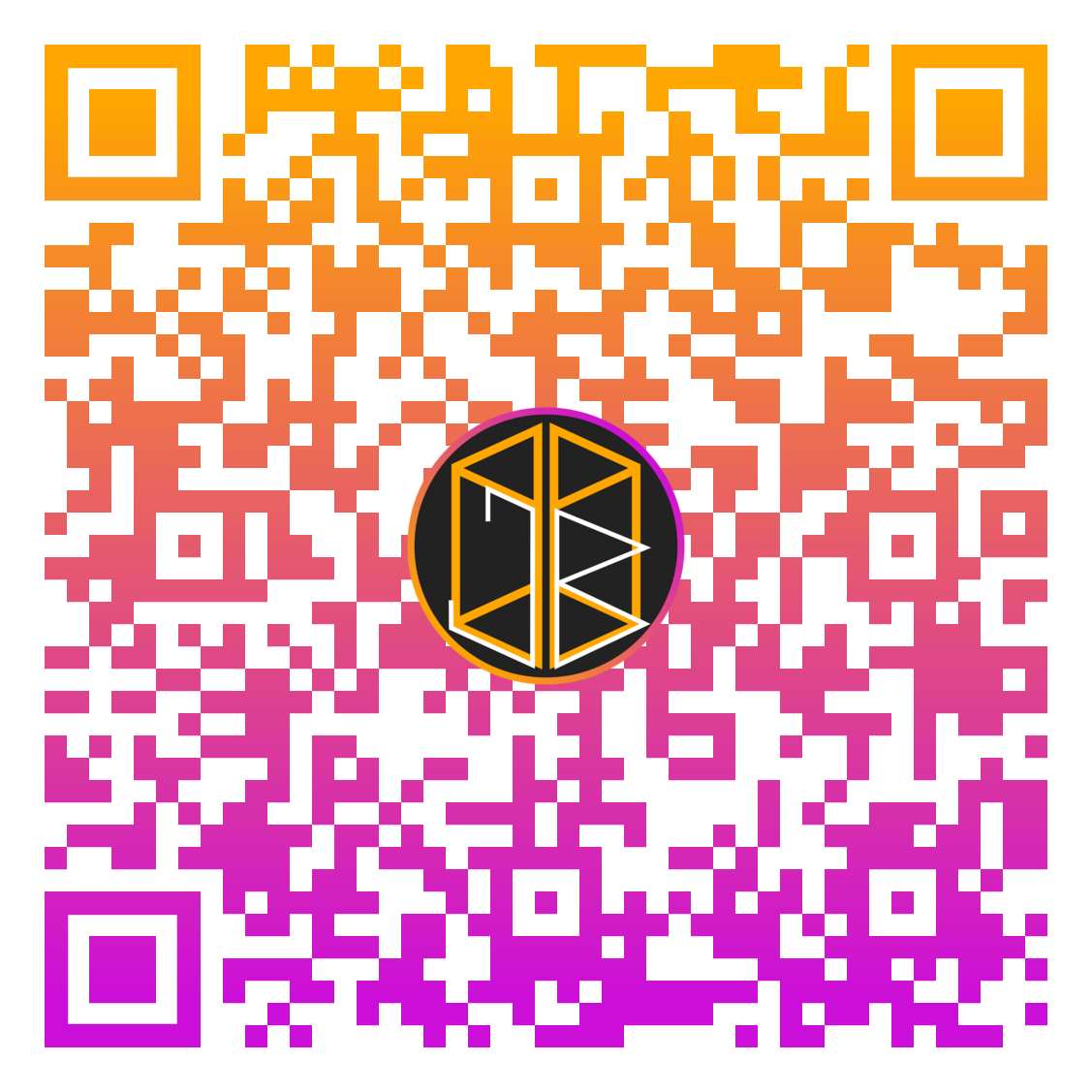